Sakura

![[CSDL - Bài Giải Mẫu] Bữa Cuối Cùng Stars7](https://2img.net/i/itest/ranks/stars/stars7.gif)

Thú CƯng : ![[CSDL - Bài Giải Mẫu] Bữa Cuối Cùng I-hate-Cats-icon](https://2img.net/h/icons.iconarchive.com/icons/benoit-bender/a-dogs-life/48/I-hate-Cats-icon.png) 
Số bài viết : 1124 Điểm : 1688 Được cảm ơn : 35 Ngày sinh : 03/11/1990 Tham gia ngày : 16/03/2010 Tuổi : 33 Đến từ : Bình Dương Ngề nghiệp : IT Student
![[CSDL - Bài Giải Mẫu] Bữa Cuối Cùng Empty](https://2img.net/i/fa/empty.gif) | Tiêu đề: [CSDL - Bài Giải Mẫu] Bữa Cuối Cùng 3/12/2010, 20:45 | |
| Đây là bài mình mới làm, ngắn hơn nhiều rồi, Khi thi, nếu được thì bỏ bớt finally đi cho nhẹ, bỏ bớt phần Design cũng ko sao. Code: - Code:
-
/** * @(#)FrameQuanLySach0.java * * FrameQuanLySach0 application * * @author: Nicholas Tse * @version 1.00 2010/12/3 */ import java.awt.event.*; import javax.swing.*; import java.sql.*; import java.awt.*; public class FrameQuanLySach0 extends JFrame implements ActionListener { JLabel lbGioiThieu; JLabel lbMaLoai; JLabel lbTenLoai; JComboBox cbMaLoai; JTextField txtMaLoai; JTextField txtTenLoai; JButton btnThem; JButton btnXoa; JButton btnSua; JButton btnThoat; JPanel pnlGioiThieu; JPanel pnlNhap; JPanel pnlButton; JPanel pnlMain; JOptionPane dialog = new JOptionPane(); static int stt = 0; static String [][]data = new String[100][2]; static int sttSach = 0; static String []dataSach = new String[100]; static int sttMaLoai = 0; static String []danhsachMaLoai = new String[100]; public FrameQuanLySach0(String title) { setTitle(title); setBounds(400, 200, 400, 100); setDefaultCloseOperation(EXIT_ON_CLOSE); setResizable(false); lbGioiThieu = new JLabel("Quan Ly Sach"); pnlGioiThieu = new JPanel(new GridLayout(1, 1)); pnlGioiThieu.add(lbGioiThieu); lbMaLoai = new JLabel("Ma Loai"); txtMaLoai = new JTextField(8); cbMaLoai = new JComboBox(); cbMaLoai.addActionListener(this); lbTenLoai = new JLabel("Ten Loai"); txtTenLoai = new JTextField(8); pnlNhap = new JPanel(new GridLayout(1, 5)); pnlNhap.add(lbMaLoai); pnlNhap.add(txtMaLoai); pnlNhap.add(cbMaLoai); pnlNhap.add(lbTenLoai); pnlNhap.add(txtTenLoai); btnThem = new JButton("Them"); btnThem.addActionListener(this); btnXoa = new JButton("Xoa"); btnXoa.addActionListener(this); btnSua = new JButton("Sua"); btnSua.addActionListener(this); btnThoat = new JButton("Thoat"); btnThoat.addActionListener(this); pnlButton = new JPanel(new GridLayout(1, 4)); pnlButton.add(btnThem); pnlButton.add(btnXoa); pnlButton.add(btnSua); pnlButton.add(btnThoat); pnlMain = new JPanel(new BorderLayout()); pnlMain.add(pnlGioiThieu, BorderLayout.NORTH); pnlMain.add(pnlNhap, BorderLayout.CENTER); pnlMain.add(pnlButton, BorderLayout.SOUTH); this.add(pnlMain); LoadData(); } //Main method public static void main(String[] args) { FrameQuanLySach0 sach = new FrameQuanLySach0("Quan Ly Sach"); sach.setVisible(true); } //Xoa het du lieu cu de load du lieu moi len private void ClearData() { stt = 0; for(int i = 0; i < 100; i++) { for(int j = 0; j < 2; j++) { if(data[i][j] != "") { data[i][j] = ""; } } } cbMaLoai.removeAllItems(); } //Kiem tra ben bang SACH, neu con MALOAI thi ko duoc xoa private boolean SearchMa(String ma) { for(int i = 0; i < sttSach; i++) { if(ma.equals((Object)dataSach[i])) { return true; } } return false; } //Phan ket noi va tuong tac voi CSDL private void ConnectToODBC() { try { Class.forName("sun.jdbc.odbc.JdbcOdbcDriver"); } catch(ClassNotFoundException e) { dialog.showMessageDialog(this, "Driver not found", "Error", JOptionPane.ERROR_MESSAGE); } } private Connection CreateConnection() { Connection con = null; try { String url = "jdbc:odbc:Driver=Microsoft Access Driver (*.mdb);DBQ=QUANLYSACHDB.MDB;readonly=false"; con = DriverManager.getConnection(url,"",""); } catch(Exception e) { dialog.showMessageDialog(this, "Khong The Ket Noi", "Error", JOptionPane.ERROR_MESSAGE); } return con; } private void ExecuteUpdate(String query) { ConnectToODBC(); Connection con = null; Statement stm = null; try { con = CreateConnection(); stm = con.createStatement(); stm.executeUpdate(query); } catch(SQLException s) { dialog.showMessageDialog(this, "Khong The Ket Noi", "Error", JOptionPane.ERROR_MESSAGE); } finally { try { stm.close(); con.close(); } catch(SQLException e) { dialog.showMessageDialog(this, "Khong The Dong Cac Ket Noi", "Error", JOptionPane.ERROR_MESSAGE); } } } private void LoadData() { ConnectToODBC(); Connection con = null; Statement stm = null; ResultSet rst = null; try { con = CreateConnection(); stm = con.createStatement(); String query = "Select * From tblLOAISACH Where XOAMALOAI ='0'"; rst = stm.executeQuery(query); ClearData(); while(rst.next()) { String ma = rst.getString(1); String ten = rst.getString(2); data[stt][0] = ma; data[stt][1] = ten; cbMaLoai.addItem((Object)ma); stt++; } } catch(SQLException s) { dialog.showMessageDialog(this, "Khong The Ket Noi", "Error", JOptionPane.ERROR_MESSAGE); } finally { try { rst.close(); stm.close(); con.close(); } catch(SQLException e) { dialog.showMessageDialog(this, "Khong The Dong Cac Ket Noi", "Error", JOptionPane.ERROR_MESSAGE); } } } private void Add(String ma, String ten) { String query = "Insert Into tblLOAISACH Values('" + ma + "', '" + ten + "', '0')"; ExecuteUpdate(query); } private void Del(String ma) { String query = "Update tblLOAISACH Set XOAMALOAI='1' Where MALOAI='" + ma + "'"; ExecuteUpdate(query); } private void Edit(String ma, String ten) { String query = "Update tblLOAISACH Set TENLOAI='" + ten + "' Where MALOAI='" + ma + "'"; ExecuteUpdate(query); } private void LoadDataSach() { ConnectToODBC(); Connection con = null; Statement stm = null; ResultSet rst = null; try { con = CreateConnection(); stm = con.createStatement(); String query = "Select MALOAI From tblSACH"; rst = stm.executeQuery(query); while(rst.next()) { dataSach[sttSach] = rst.getString(1); sttSach++; } } catch(Exception e) { System.out.println (e.getMessage()); } finally { try { rst.close(); stm.close(); con.close(); } catch(Exception e) { System.out.println (e.getMessage()); } } } //Ket thuc phan tuong tac voi CSDL public void actionPerformed(java.awt.event.ActionEvent e) { if(e.getSource() == cbMaLoai) { int index = cbMaLoai.getSelectedIndex(); System.out.println ("Index = " + index); if(index >= 0) { txtMaLoai.setText(data[index][0]); txtTenLoai.setText(data[index][1]); } } if(e.getSource() == btnThem) { String ma = txtMaLoai.getText(); String ten = txtTenLoai.getText(); if(ma.length() != 0 && ten.length() != 0) { Add(ma, ten); dialog.showMessageDialog(this, "Them thanh cong", "OK!", JOptionPane.INFORMATION_MESSAGE); LoadData(); } else { dialog.showMessageDialog(this, "Khong the them", "Error", JOptionPane.ERROR_MESSAGE); } } else if(e.getSource() == btnXoa) { String ma = String.valueOf(cbMaLoai.getSelectedItem()); if(SearchMa(ma) == false) { Del(ma); dialog.showMessageDialog(this, "Xoa thanh cong", "OK!", JOptionPane.INFORMATION_MESSAGE); LoadData(); } else { dialog.showMessageDialog(this, "Khong the xoa", "Error", JOptionPane.ERROR_MESSAGE); } } else if(e.getSource() == btnSua) { String ma = String.valueOf(cbMaLoai.getSelectedItem()); String ten = txtTenLoai.getText(); if(ten.length() != 0) { Edit(ma, ten); dialog.showMessageDialog(this, "Sua thanh cong", "OK!", JOptionPane.INFORMATION_MESSAGE); LoadData(); } else { dialog.showMessageDialog(this, "Khong the xoa", "Error", JOptionPane.ERROR_MESSAGE); } } else if(e.getSource() == btnThoat) { System.exit(1); } } } Link bài Full + CSDL Access: [You must be registered and logged in to see this link.] |
|
bubupro.gdty
Member Năng Động

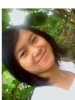
Thú CƯng : ![[CSDL - Bài Giải Mẫu] Bữa Cuối Cùng Turtle-icon](https://2img.net/h/icons.iconarchive.com/icons/visualpharm/animals/48/Turtle-icon.png) 
Số bài viết : 118 Điểm : 123 Được cảm ơn : 0 Ngày sinh : 01/04/1990 Tham gia ngày : 02/04/2010 Tuổi : 34 Đến từ : Gia Lai Ngề nghiệp : student Chăm ngôn : to be or not to be
![[CSDL - Bài Giải Mẫu] Bữa Cuối Cùng Empty](https://2img.net/i/fa/empty.gif) | Tiêu đề: Re: [CSDL - Bài Giải Mẫu] Bữa Cuối Cùng 3/12/2010, 23:06 | |
| bóc tem nà  hjhj |
|